In today’s fast-paced software landscape, conducting a thorough due diligence assessment of Python-based projects is crucial for building and maintaining secure, reliable, and compliant systems. This guide consolidates key practices for evaluating Python applications across three critical domains: Security, Licensing, and Code Ownership
Security
Security in Python projects goes beyond the language’s flexibility and extensive standard library. While Python enables rapid development and offers a rich ecosystem of third-party packages, additional measures are necessary to protect applications effectively.
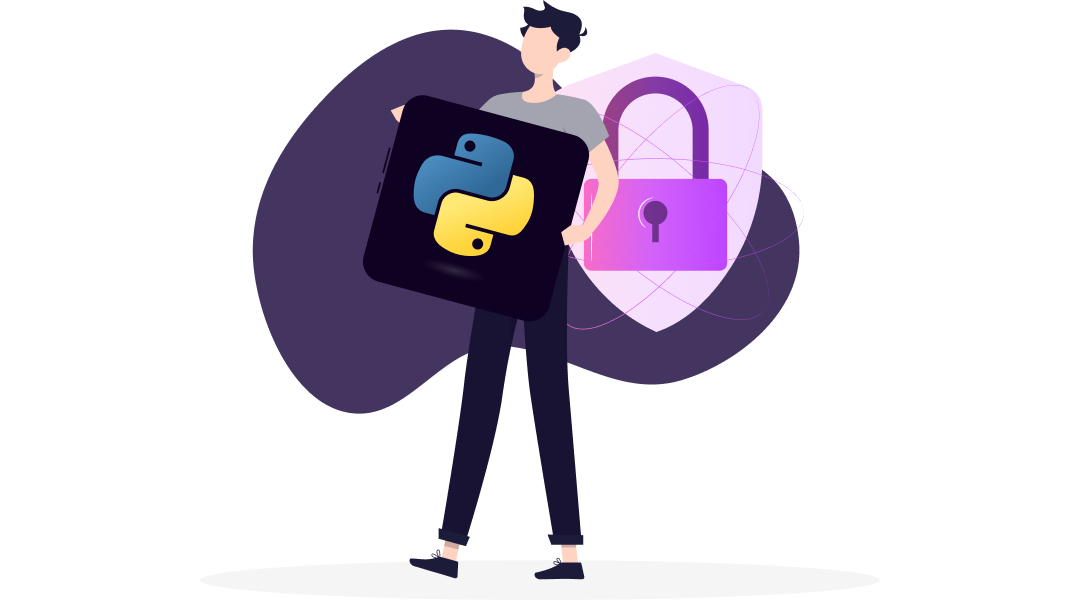
1. Code-Related Security Measures
1.1 General Security Measures
- Input Validation
- Validate and sanitize all user inputs to prevent injection attacks (e.g., SQL Injection, XSS, Command Injection) as outlined in OWASP A03:2021.
- Use frameworks or libraries with built-in validation and escaping mechanisms (e.g., Django’s form validation, WTForms for Flask, Pydantic for FastAPI).
- Incorporate type checking (e.g., using mypy) to catch type-related vulnerabilities early.
- Use Static Analysis Tools
- Tools like Bandit, Pylint, Flake8, Ruff, and SonarQube can detect a wide range of security issues and code hygiene problems.
- Integrate these tools into the CI/CD pipeline for continuous feedback on code quality and security.
- Prevent Insecure Deserialization or Code Injection
- Avoid using pickle for untrusted data to prevent remote code execution; use safer serialization formats such as JSON or YAML.
- Refrain from using eval() or exec() with untrusted inputs. If you must parse data, use safe alternatives like ast.literal_eval().
- Secure Python’s Runtime Environment
- Use virtual environments to isolate project dependencies and reduce the risk of Python path manipulation.
- Avoid dynamically importing modules from untrusted sources.
- Carefully handle file operations (os.system, subprocess.call, etc.) and consider modules like shlex for argument parsing to prevent command injection.
1.2 Framework-Related Security Measures
- Django
- XSS Protection: Rely on Django’s template engine, which auto-escapes variables by default.
- CSRF Protection: Keep CSRF middleware enabled; verify that every form submits a valid token.
- SQL Injection Prevention: Use Django’s ORM or parameterized queries; never concatenate raw queries with user input.
- Authentication & Authorization: Configure Django’s authentication system properly to prevent privilege escalation.
- Flask
- CSRF: Integrate libraries like Flask-WTF for CSRF protection.
- Session Management: Configure secure sessions (e.g., set SESSION_COOKIE_SECURE in production).
- Security Libraries: Use MarkupSafe or similar packages for escaping.
- FastAPI
- Validation: Leverage Pydantic for robust data validation and type enforcement to mitigate injection risks.
- OAuth2 / JWT: Ensure token-based auth is properly configured and tokens are securely stored and verified.
- ORM Usage: If using SQLAlchemy, rely on parameterized queries or safe query-building methods.
2. Dependency-Related Security Measures
Python’s package ecosystem (PyPI) provides a vast selection of third-party libraries but requires vigilant oversight to mitigate vulnerabilities.
2.1 Audit and Monitor Dependencies
- Use tools like pip-audit, Safety, or Dependabot to identify known CVEs.
- Subscribe to security advisories or monitor mailing lists for critical packages.
2.2 Regular Updates
- Keep frameworks and libraries up-to-date to address known vulnerabilities promptly.
- Pin dependencies to specific versions (via requirements.txt or pyproject.toml) for reproducible builds, but periodically review pinned versions to avoid accumulating technical debt.
2.3 Use Trusted Repositories
- Host an internal PyPI mirror if necessary, or rely on official mirrors.
- Verify package integrity (e.g., using pip’s —require-hashes mode).
2.4 Minimize Dependency Tree
- Remove unused or redundant libraries.
- Each additional dependency can introduce vulnerabilities or licensing complications.
3. Importance of Penetration Testing
Static analysis and dependency management tools cannot guarantee complete security coverage. Penetration testing simulates real-world attacks to uncover hidden vulnerabilities.
3.1 Simulate Attack Scenarios
- Consider common issues: Broken Access Control (OWASP A01:2021), Security Misconfigurations (OWASP A05:2021), template injections, and insecure subprocess usage.
3.2 Include Infrastructure
- Assess the underlying servers, load balancers, and database configurations.
- Check for proper HTTPS setups, valid SSL certificates, and secure network configurations.
3.3 Validate Configuration & Deployment
- Ensure secrets (API keys, database credentials) are not hardcoded or committed to version control.
- Confirm that containers, virtual environments, or other deployment structures isolate services correctly.
License Compliance
Python-based projects often rely on a mix of open-source libraries from PyPI and other sources. Understanding license obligations is essential to avoid legal and operational risks.
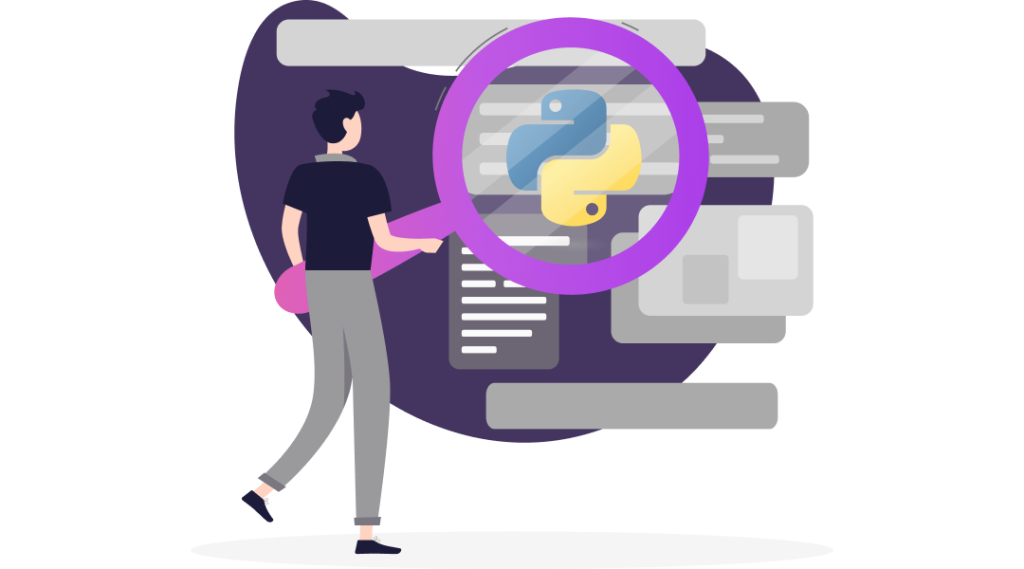
Detecting Licenses and Ensuring Compliance
1. License Detection
- Use tools like pip-licenses, LicenseFinder, or custom scripts to scan direct and transitive dependencies.
- Monitor for packages that may have changed their license terms over time.
2. Compliance Measures
- Maintain a license compatibility matrix to ensure you are not combining libraries with conflicting terms.
- Integrate automated license scanning into your CI/CD pipeline; reject changes that introduce incompatible licenses.
3. Flag Critical Licenses
- Permissive Licenses (Apache 2.0, MIT, BSD): Generally straightforward for commercial use.
- Restrictive Licenses (GPL, AGPL): May require you to open-source your code if you distribute software containing these dependencies. Review obligations carefully.
Code Ownership & Governance
Proper governance ensures a Python codebase remains maintainable, resilient to turnover, and aligned with best practices.
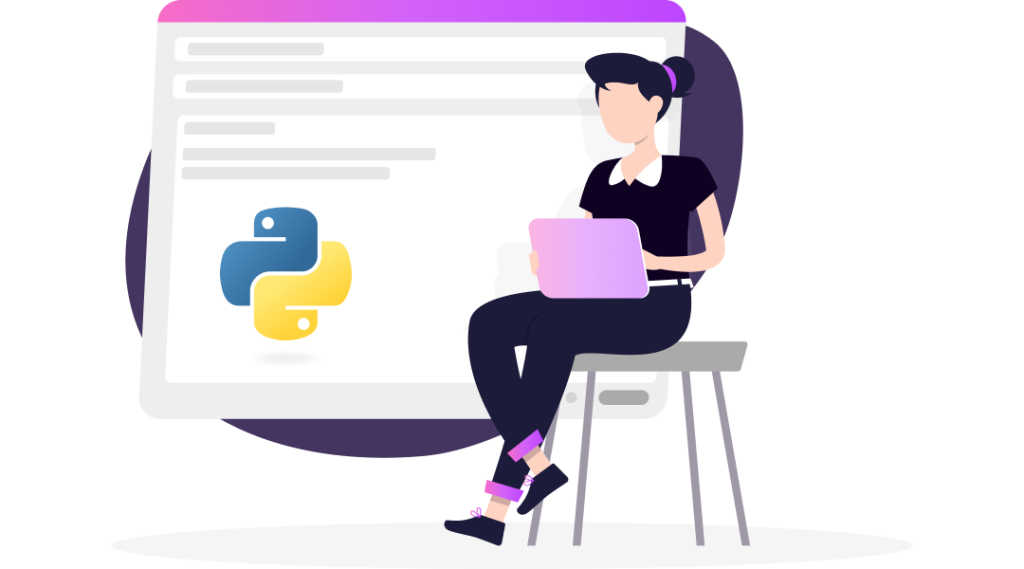
1. Detecting Bad Practices in Code Ownership
1.1 Indicators of Poor Code Ownership
- Ex-Developer Concentration: Large portions of the codebase come from contributors who are no longer active.
- Sparse Documentation: Outdated or missing docstrings, README files, or generated docs (e.g., Sphinx).
- Low Codebase Distribution: Most commits come from a small group, increasing the “bus factor” risk.
1.2 Code Quality Metrics
- Track test coverage using coverage.py or tox.
- Assess complexity with radon (e.g., McCabe Complexity).
- Enforce coding standards with Flake8, Black, isort, or Pylint.
2. Tools for Assessment
- Version Control Analysis: Tools like SonarQube can combine commit data, static analysis, and code quality metrics in one dashboard.
- Code Review Policies: Enforce peer reviews, track developer participation, and encourage knowledge sharing to reduce silos.
3 Mitigation Strategies
3.1 Knowledge Transfer
- Facilitate pair or mob programming sessions to distribute expertise.
- Keep documentation current—docstrings, architectural decision records (ADRs), and wikis.
3.2 Code Rotation
- Rotate ownership of modules or features so multiple team members understand critical components.
- Involve junior developers early in high-risk areas to reduce reliance on single experts.
3.3 Monitor Turnover Risks
- .Identify critical contributors whose departure could severely impact the project.
- Develop onboarding processes that accelerate new developers’ familiarity with core areas.
Conclusion
Performing a due diligence assessment for Python-based projects requires a holistic view that spans security, license compliance, and code governance. By integrating the recommendations below into regular assessments, you can mitigate risks early, reduce technical debt, and maintain a competitive edge:
- Security: Implement proactive measures—robust input validation, safe deserialization (avoid pickle for untrusted data), secure framework configurations, and regular penetration testing.
- License Compliance: Continuously detect and document license obligations to prevent legal pitfalls; ensure automated scanning of any new or updated dependencies.
- Code Ownership & Governance: Encourage balanced contributions, maintain thorough documentation, enforce code reviews, and foster knowledge sharing to minimize the “bus factor” risk.
A well-governed, secure, and license-compliant Python environment is the backbone of sustainable software development. By incorporating these best practices, organizations can build resilient, high-quality Python applications that stand the test of time.