In today’s fast-paced software landscape, conducting a thorough due diligence assessment of PHP-based projects is essential for building and maintaining secure, reliable, and compliant systems. This guide consolidates key practices for evaluating PHP applications across three critical domains: Security, Licensing, and Code Ownership
Security
PHP’s web-centric nature, combined with its extensive package ecosystem, makes it particularly vulnerable to common web exploits if not configured and coded securely. Modern PHP offers many safeguards, but additional measures are necessary to protect applications effectively.
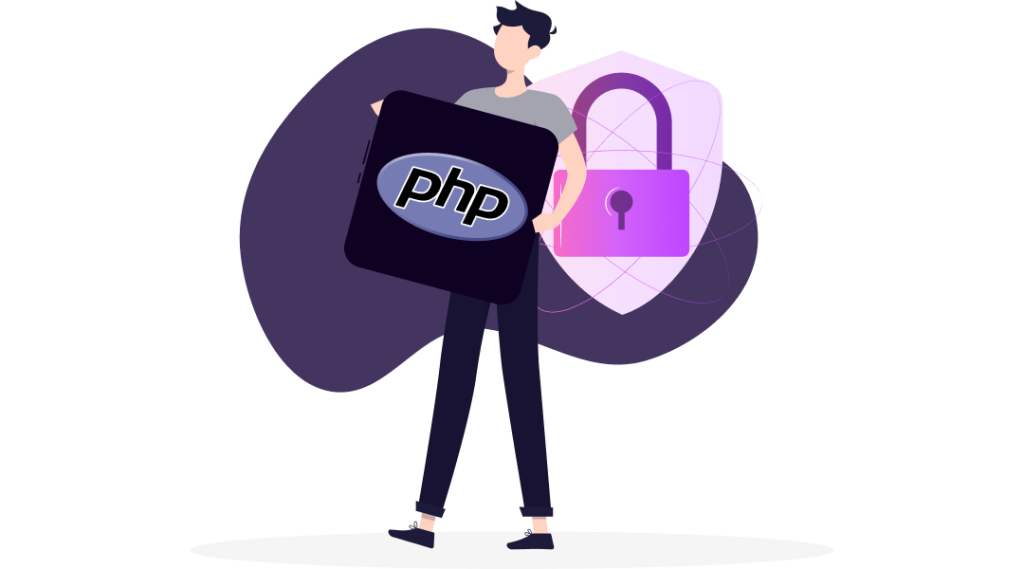
1. Code-Related Security Measures
1.1 General Security Measure
- Input Validation & Sanitization
- Use OWASP A03:2021-Injection as a reference.
- Leverage PHP’s built-in filter_* functions (e.g., filter_input()) to validate and sanitize user input.
- Use HTMLPurifier for HTML content sanitization.
- Use type declarations (PHP 7+) for stronger type safety.
- Apply htmlspecialchars() or equivalent output encoding to prevent XSS.
- Validate file uploads carefully (e.g., file type, size) and store them outside the webroot.
- Static Analysis & Code Quality Tools
- PHP_CodeSniffer: Enforce PSR standards and check for common security or style issues.
- PHPStan or Psalm: Perform static analysis and type checking.
- RIPS or similar security-focused scanners for deeper analysis of PHP-specific vulnerabilities.
- Integrate these tools into your CI/CD pipeline for continuous feedback.
- Prevent Code Injection
- Avoid dynamic execution functions like eval(), create_function(), and untrusted unserialize().
- Use parameterized queries (PDO or MySQLi) for all database interactions to mitigate SQL injection.
- Carefully escape shell command parameters (preferably avoid functions like exec(), shell_exec() with user input).
- Use safe alternatives for potentially dangerous functions (e.g., password_hash() instead of manual cryptography).
- Error Handling & Logging
- Disable detailed error display in production; log errors to a secure location instead.
- Use appropriate logging levels (error, warning, info) and rotate logs to avoid exposing sensitive data.
- Session & Password Management
- Configure secure session settings (e.g., session.cookie_secure, session.cookie_httponly).
- Regenerate session IDs after login to prevent session fixation.
- Never store passwords in plain text—use password_hash() (bcrypt, Argon2) and password_verify().
- Implement secure password reset mechanisms (e.g., time-limited, token-based).
- File Handling
- Validate file paths to prevent directory traversal (ensure paths are whitelisted or sanitized).
- Store uploaded files outside the web-accessible directory and use randomized file names.
- Apply appropriate permissions to uploaded files.
1.2 Framework-Related Security Measures
Popular PHP frameworks each provide robust security features—configure them properly to maximize protection:
- Laravel
- Enable built-in CSRF tokens with forms.
- Use Eloquent ORM or Query Builder with parameterized queries.
- Implement auth middleware for role-based access control.
- Configure session handling securely (e.g., encryption, secure cookies).
- Use Form Request Validation to centralize validation logic.
- Symfony
- Leverage the Security Components for authentication, authorization, and CSRF protection.
- Use Doctrine ORM securely with parameterized queries.
- Implement access control rules in security.yaml.
- Utilize Symfony’s form validation to sanitize inputs.
- CodeIgniter
- Enable built-in XSS filtering and security helper functions.
- Use the Query Builder or parameterized queries for database interactions.
- Implement secure session management (e.g., encryption, secure cookies).
- Configure file upload handling to restrict file types and sizes.Use version.
2. Dependency-Related Security Measures
PHP’s Composer ecosystem (Packagist) offers convenience and flexibility but requires careful management:
2.1 Audit Dependencies
- Run composer audit or use security-checker (e.g., Roave Security Advisories) to detect known vulnerabilities.
- Monitor official PHP Security Advisories and relevant mailing lists.
2.2 Update Strategy
- Use version constraints (composer.json) sensibly to receive security patches without accidentally upgrading to breaking versions.
- Employ tools like Dependabot for automated pull requests on dependency updates
- Regularly align dependencies with your PHP version—ensure core PHP is also kept up to date.
2.3 Minimize Attack Surface
- Regularly audit installed Composer packages; remove unused dependencies.
- Use minimal dependencies in production, and optimize autoloading (composer dump-autoload –optimize).
- Restrict dynamic includes and verify file paths to avoid malicious loadable scripts.
3. Importance of Penetration Testing
Even with static analysis and diligent dependency management, real-world attack simulations can uncover overlooked vulnerabilities:
- Common Focus Areas
- SQL Injection: Test forms and API endpoints with malicious inputs.
- Cross-Site Scripting (XSS): Check both reflected and stored XSS in user input fields.
- File Inclusion & Upload Vulnerabilities: Confirm that file uploads and includes are strictly controlled.
- Session Security: Validate session handling for fixation or hijacking scenarios.
- Command Injection: Inspect any feature running shell commands or external processes.
License Compliance
PHP’s Composer-based package management often results in many indirect (transitive) dependencies. Understanding license obligations is key to avoiding legal pitfalls.
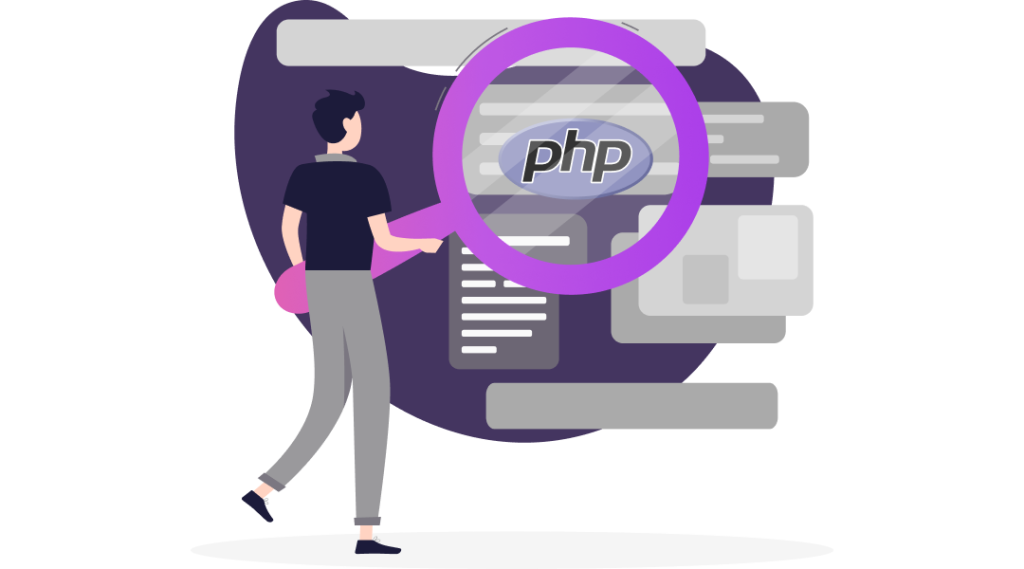
Detecting Licenses and Ensuring Compliance
- License Detection
- Run composer licenses (Requires external plugin) or use specialized license-checker tools.
- Review both direct and transitive dependencies to identify all license types (MIT, GPL, BSD, etc.).
- Regularly audit the composer.json and composer.lock for any license changes.
- Compliance Measures
- Maintain a license compatibility matrix to ensure you do not violate your organization’s legal or policy constraints.
- Integrate automated license checks into CI/CD pipelines; flag or block merges that introduce incompatible licenses.
- Permissive: MIT, BSD, Apache (generally easier for commercial use).
- Copyleft: GPL, LGPL (review obligations carefully, as they may require distributing source code).
- Custom: Verify the terms for lesser-known or privately licensed packages.
Code Ownership & Governance
Proper code governance ensures maintainability, reduces the “bus factor,” and promotes best practices.
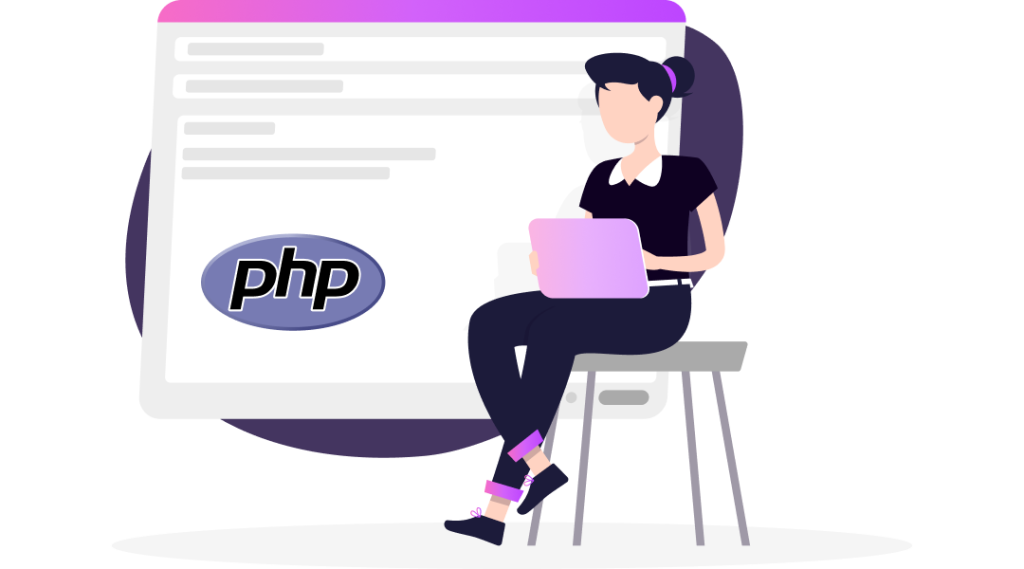
1. Detecting Bad Practices in Code Ownership
1.1 Code Quality Indicators
- Excessive Complexity: High cyclomatic complexity or deeply nested logic.
- Poor Adherence to PSR Standards: Mixed coding styles, missing namespaces.
- Sparse Documentation: Missing or outdated PHPDoc, READMEs, or architectural notes.
- Inconsistent Namespace Usage or folder structure.
- Weak Error Handling Patterns: Use of @ suppression, incomplete exception handling.
1.2 Knowledge Distribution
- Monitor the “bus factor” by identifying modules with only one contributor.
- Track documentation coverage and code review participation.
- Encourage cross-training to reduce reliance on a single developer.
2. Tools for Assessment
2.1 Static Analysis [[Is not that repetition from Static Analysis & Code Quality Tools]]
- PHPMD (PHP Mess Detector) for detecting code smells and complexity.
- PHPUnit for test coverage.
- PHP CS Fixer or PHP_CodeSniffer for automated style checking.
2.2 Version Control & Code Review
- Use Git for version control and structured branching.
- Enforce mandatory code reviews to distribute knowledge and maintain quality.
3. Mitigation Strategies
3.1 Knowledge Management
- Maintain comprehensive PHPDoc with clear function- and class-level comments.
- Use Architecture Decision Records (ADRs) to document important design choices.
- Regular code review and pair programming sessions.
3.2 Code Rotation & Onboarding
- Rotate developers through different modules or components.
- Onboard junior developers early to critical areas to avoid single-expert silos.
Conclusion
A thorough due diligence assessment for PHP-based projects requires a well-rounded approach spanning security, license compliance, and governance. Key takeaways include:
- Security
- Validate, sanitize, and encode all user inputs.
- Adopt framework-specific security features (Laravel, Symfony, CodeIgniter) and enforce best practices (prepared statements, session hardening, etc.).
- Maintain strict Composer dependency management, with regular audits and updates.
- Conduct penetration testing to uncover hidden vulnerabilities.
- License Compliance
- Continuously monitor both direct and transitive dependencies for license changes or conflicts.
- Use automated checks and maintain a compatibility matrix to avoid legal pitfalls.
- Code Ownership & Governance
- Enforce coding standards, documentation, and code reviews to maintain quality and distribute knowledge.
- Implement static analysis tools and encourage collaborative development to reduce reliance on single contributors.
By integrating these recommendations into ongoing development and deployment practices, you can significantly reduce risk, maintain legal and operational integrity, and ensure the long-term success of your PHP projects. A well-governed, secure, and license-compliant environment is the cornerstone of sustainable software development.