In today’s fast-paced software landscape, conducting a thorough due diligence assessment of JavaScript-based projects is essential for building and maintaining secure, reliable, and compliant systems. This guide consolidates key practices for evaluating JavaScript applications across three critical domains: Security, Licensing, and Code Ownership
Security
JavaScript’s dynamic nature, popularity in both frontend and backend development, and extensive package ecosystem (npm/yarn) make it a frequent target for vulnerabilities. Beyond the language’s built-in features, additional measures are necessary to protect applications effectively.
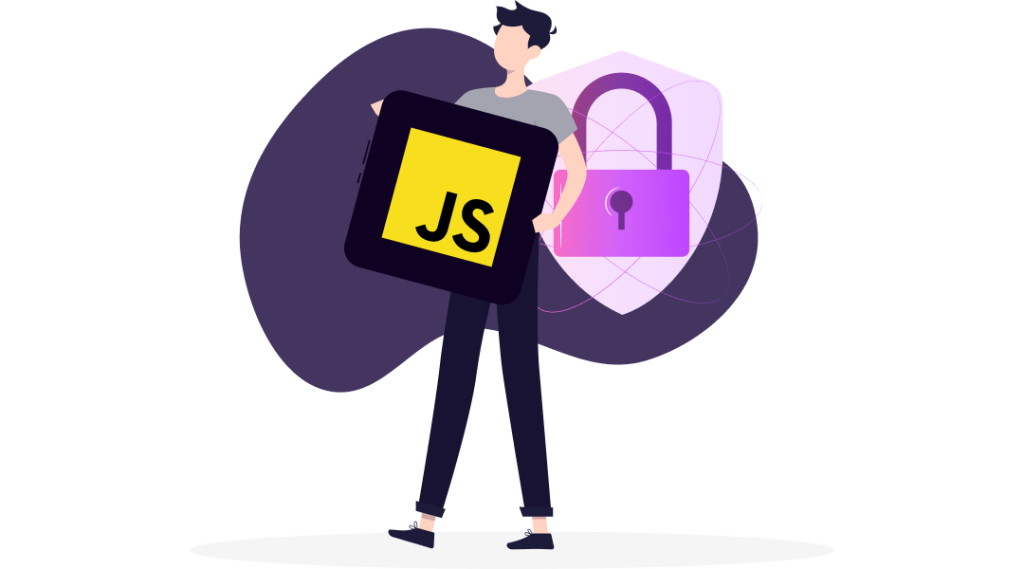
1. Code-Related Security Measures
1.1 General Security Measures
- Input Validation & Sanitization
- Validate and sanitize user inputs to prevent injection attacks (e.g., XSS, SQL Injection) as outlined in OWASP A03:2021.
- Use libraries like validator.js, DOMPurify, Joi, or Zod for structured data validation and HTML sanitization.
- Adopt runtime type checking (or TypeScript for compile-time checking) to reduce type-related security flaws.
- Static Analysis Tools
- Integrate ESLint, SonarQube, JSHint, or StandardJS in your CI/CD pipeline to detect code quality and security issues.
- Consider security-focused plugins (e.g., eslint-plugin-security, eslint-plugin-n) for additional checks.
- Avoid Dangerous APIs & Code Injection
- Refrain from using eval(), new Function(), or string-based arguments in setTimeout/setInterval.
- Parse JSON data using JSON.parse() instead of eval().
- Sanitize template literals or dynamic expressions that incorporate user input.
- Implement a Content Security Policy (CSP) to restrict the sources from which scripts, styles, and other resources can be loaded.
- Secure Environment Variables
- Store sensitive credentials (API keys, tokens) in environment variables using tools like dotenv.
- Exclude .env files from source control via .gitignore.
- Ensure production builds do not expose secrets in client-facing code.
1.2 Framework-Related Security Measures
JavaScript frameworks each introduce unique security considerations. Properly configuring these tools is crucial:
1. Frontend Frameworks
- React
- Sanitize content passed to dangerouslySetInnerHTML and use it sparingly.
- Leverage React’s built-in safeguards against XSS by escaping JSX expressions automatically.
- Be cautious with side effects in hooks like useEffect.
- Vue.js
- Use Vue’s built-in sanitization for bound data.
- Limit or sanitize inputs passed to directives like v-html.
- Angular
- Enable strict CSP to block unauthorized scripts.
- Use DomSanitizer for any user-supplied content.
- Configure XSRF/CSRF tokens when making HTTP calls.
2. Backend Frameworks
- Express
- Validate inputs with libraries like express-validator.
- Set secure HTTP headers using helmet.js.
- Implement rate limiting (e.g., express-rate-limit) to thwart brute force attacks.
- Next.js / Nuxt.js
- Configure secure response headers in server-side API endpoints.
- Use static site generation (SSG) or server-side rendering (SSR) carefully, avoiding dynamic code injection.
2. Dependency-Related Security Measures
JavaScript projects often depend on numerous packages managed by npm or yarn. Proper oversight of these dependencies is critical.
2.1. Audit Dependencies
- Use npm audit, Dependabot, or Retire.js to identify known vulnerabilities (including transitive dependencies).
- Monitor vulnerability advisories from the Node Security Project and GitHub Security Advisories.
2.2. Regular Updates
- Keep dependencies up-to-date, using tools like Dependabot for automated pull requests.
- Use semver ranges mindfully to avoid breaking changes from major version upgrades.
- Pin dependencies in package-lock.json or yarn.lock for deterministic builds.
2.3. Minimize Dependencies
- Remove unused or redundant packages with depcheck or webpack-bundle-analyzer.
- Favor lightweight, single-purpose libraries over large, all-in-one utilities.
2.4. Verify Package Integrity
- Use npm ci in CI pipelines for deterministic installs.
- Leverage npm’s integrity checks (SHA-256 hashes) to ensure packages haven’t been tampered with.
3. Importance of Penetration Testing
While static analysis and dependency audits address many security concerns, penetration testing simulates real-world attacks to identify potential runtime vulnerabilities:
3.1. Simulate Attack Scenarios
- Test for XSS, SQL Injection, SSRF, and misconfigured CORS policies.
- Ensure client-side logic and session tokens are not susceptible to replay or interception.
3.2. Infrastructure Security
- Evaluate hosting services (AWS, Azure, Vercel, etc.) for proper firewall rules, secure SSL/TLS configurations, and limited public exposure.
- Check CDN configurations for potential data leaks or caching misconfigurations.
License Compliance
The JavaScript ecosystem’s heavy reliance on external libraries means that license obligations can become complex and potentially risky if unmanaged.
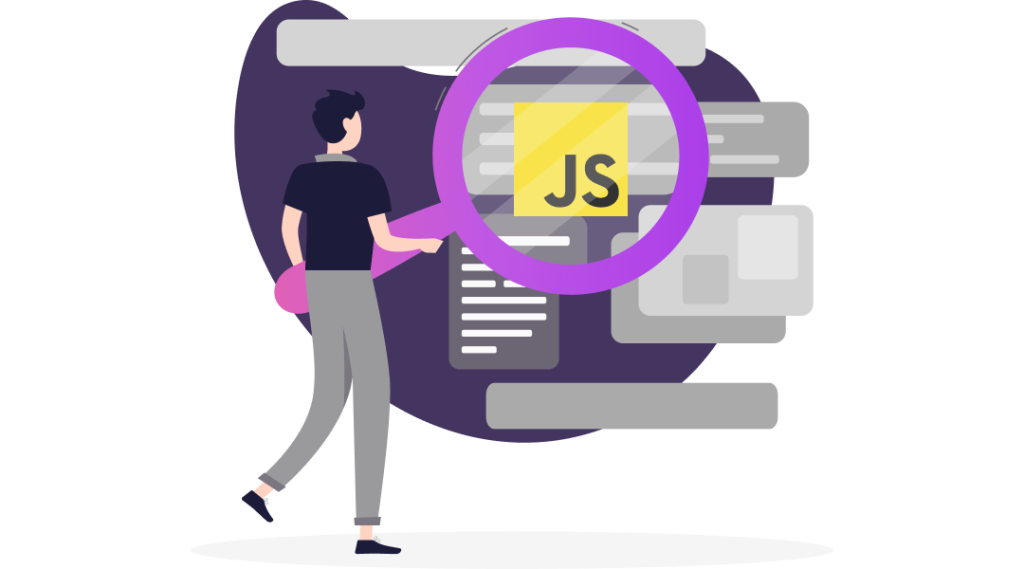
Detecting Licenses and Ensuring Compliance
1. License Detection
- Use tools like license-checker, npm-license-crawler, or Webpack License Plugin to scan both direct and transitive dependencies.
- Pay attention to dependencies that change license terms or have dual licensing models.
2. Compliance Measures
- Maintain a license compliance matrix mapping each dependency to your organization’s policy.
- Automate license scanning in your CI/CD pipeline to prevent merging code that introduces incompatible licenses.
3. Critical License Types
- Permissive (MIT, Apache 2.0): Generally favorable for commercial applications.
- Restrictive (GPL, AGPL): May require distributing your source code if included in proprietary software. Verify obligations carefully.
Code Ownership & Governance
Proper governance ensures your JavaScript codebase remains maintainable, resilient to turnover, and aligned with best practices over time.
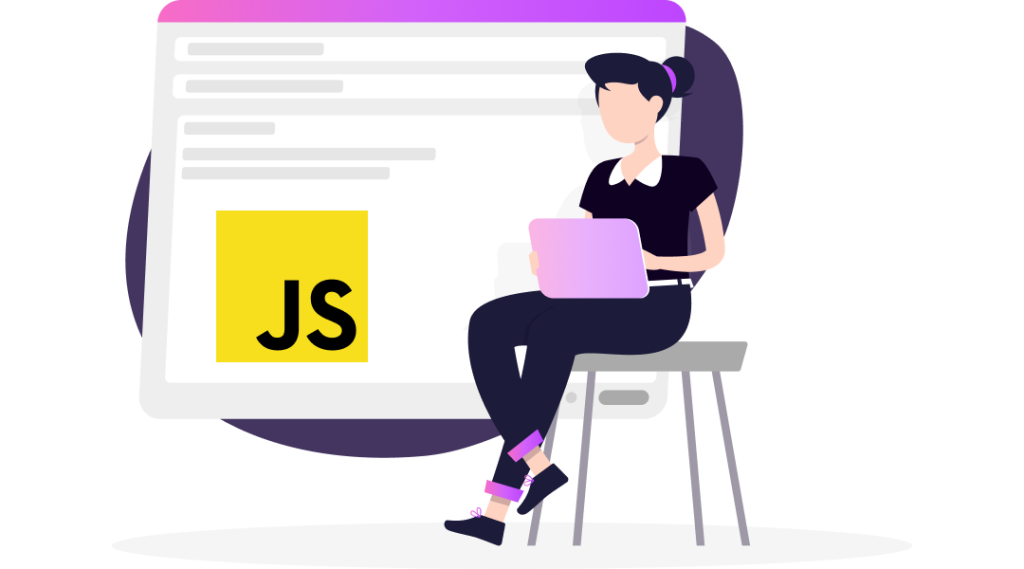
1. Detecting Bad Practices in Code Ownership
1.1 Indicators of Poor Code Ownership
- Single-Developer Dependency: Most code authored by one person, creating a “bus factor” risk.
- Sparse Documentation: Missing or outdated READMEs, lack of JSDoc or TypeDoc annotations.
- High Complexity: Deeply nested callbacks or inconsistent async patterns that reduce maintainability.
1.2 Code Quality Metrics
- Measure test coverage using tools like Jest, Mocha
- Analyze code maintainability with SonarQube or CodeClimate
- Enforce coding standards using ESLint or Prettier.
2. Tools for Assessment
2.1 Version Control Analysis
- Inspect commit histories with git log or SonarQube to identify areas with limited contributor diversity.
- Look for modules frequently touched by only one developer.
2.2 Code Review Policies
- Enforce mandatory peer reviews.
- Track participation rates to ensure knowledge sharing across teams.
3. Mitigation Strategies
3.1 Knowledge Transfer
- Document key APIs and architectural decisions using JSDoc, TypeDoc, or ADRs (Architecture Decision Records).
- Conduct regular knowledge-sharing sessions and cross-training.
3.2 Code Rotation
- Rotate feature/module ownership to avoid silos.
- Onboard junior developers early to critical areas to reduce reliance on senior staff.
3.3 Monitor Turnover Risks
- Identify critical contributors whose departure could severely impact the project.
- Plan for handovers and maintain a clear onboarding process for new developers
Conclusion
Performing a due diligence assessment for JavaScript-based projects involves a holistic approach spanning security, license compliance, and governance:
- Security
- Implement robust input validation and sanitization measures, secure your dependencies, and conduct regular penetration testing.
- Leverage framework-specific security features (React, Vue, Angular, Express, Next.js, etc.).
- License Compliance
- Continuously detect and document licenses across all dependencies.
- Enforce automated checks to prevent the introduction of incompatible or high-risk licenses.
- Code Ownership & Governance
- Encourage balanced contributions, maintain thorough documentation, and enforce code reviews to mitigate turnover risks.
- Foster a culture of knowledge sharing and collective responsibility.
By integrating these best practices into ongoing development, organizations can reduce risks, maintain high technical standards, and ensure a sustainable and competitive edge. A well-governed, secure, and legally compliant JavaScript environment forms the backbone of successful modern software development.