In today’s fast-paced software landscape, conducting a thorough due diligence assessment of Java-based projects is vital for building and maintaining secure, reliable, and compliant systems. This guide consolidates key practices for evaluating Java applications across three critical domains: Security, Licensing, and Code Ownership
Security
Security in Java projects extends beyond the language’s inherent protections. Although Java offers robust features—such as a strong type system and automatic memory management—there are numerous additional steps you must take to protect your applications effectively.
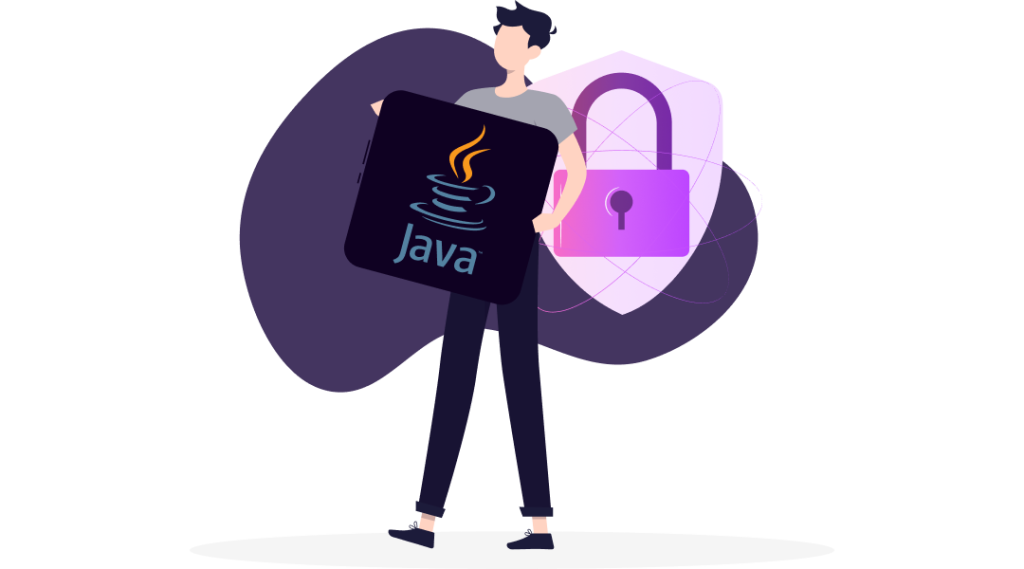
1. Code-Related Security Measures
1.1 General Security Measures
- Input Validation
- Validate and sanitize all user inputs at application boundaries to prevent Injection attacks (e.g., SQL Injection, XSS) as described in OWASP A03:2021.
- Use established libraries, such as OWASP Java Encoder or Apache Commons Validator, to handle common input validation and encoding tasks.
- Use Static Analysis Tools
- SpotBugs (FindBugs), PMD, SonarQube — these can detect a wide range of issues, from security misconfigurations to null pointer risks.
- Integrate these tools into your CI/CD pipeline for continuous feedback on code quality and security.
- Avoid Deserialization Vulnerabilities
- Uncontrolled deserialization can lead to remote code execution. Use safer alternatives (e.g., JSON) instead of Java’s native ObjectInputStream.
- If deserialization is unavoidable, configure frameworks like Jackson with strict features (DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES) to prevent unexpected data from being deserialized.
- Secure Class Loading
- The Java ClassLoader can be abused to load malicious classes if misconfigured.
- Avoid dynamically loading classes from untrusted sources, and configure security policies when necessary (e.g., when using plugins or modular architectures).
1.2 Framework-Related Security Measures
Most Java projects rely on frameworks such as Spring, Jakarta EE, Hibernate, or Micronaut. While these provide powerful features and abstractions, they also introduce unique attack vectors.
- Spring Security Vulnerabilities
- Cross-Site Scripting (XSS): Use Spring’s built-in escaping (in views like Thymeleaf or JSP with ) and avoid disabling Spring Security’s default protections.
- CSRF Protection: Ensure CSRF tokens are enabled in state-changing operations.
- Authentication & Authorization: Properly configure roles and restrict access to sensitive endpoints; misconfigurations can inadvertently open backdoors.
- SQL Injection in JPA/Hibernate
- Always use parameterized queries via JPA methods (createQuery with named parameters or createNativeQuery with positional parameters).
- Avoid concatenating user input into JPQL/HQL strings.
- Validate and sanitize data before using it in queries.
- Hibernate/JPA Entity Management
- Be cautious with lazy-loaded entities in detached contexts, which can lead to unexpected data exposure.
- Follow best practices for session management to avoid inadvertently exposing data.
2. Dependency-Related Security Measures
Modern Java projects rely on extensive dependency trees managed by Maven or Gradle. Proper oversight is crucial to prevent vulnerabilities lurking in third-party libraries.
2.1 Audit and Monitor Dependencies
- Use tools like OWASP Dependency-Check to identify known vulnerabilities.
- Track newly disclosed CVEs relevant to your dependencies.
2.2 Update Regularly
- Keep libraries up-to-date to mitigate known security flaws.
- Consider using Dependabot (GitHub) or similar tools to automate version checks.
2.3 Use a Bill of Materials (BOM)
- Adopt a BOM approach to maintain consistent, secure versions across multiple modules.
- Verify checksums (SHA-256, etc.) for downloaded artifacts, particularly those from less-trusted repositories.
2.4 Minimize Dependency Tree
- Remove unused libraries. Every additional dependency can introduce new vulnerabilities.
3. Importance of Penetration Testing
Static analysis and dependency management alone can’t guarantee complete coverage. A penetration test simulates real-world attacks to uncover overlooked vulnerabilities:
3.1 Simulate Attack Scenarios
- Common areas: Broken Access Control (OWASP A01:2021), Security Misconfigurations (OWASP A05:2021), Deserialization attacks, or misconfigured ClassLoaders.
3.2 Include Infrastructure
- Evaluate web servers, load balancers, and database connections alongside the application.
3.3 Validate Java-Specific Configurations
- Confirm that cryptographic practices (e.g., using the JCE) and the Java Security Manager are correctly implemented, if applicable.
License
Java ecosystem’s extensive use of external libraries and frameworks means that license obligations can quickly become intricate and potentially risky if not properly managed.
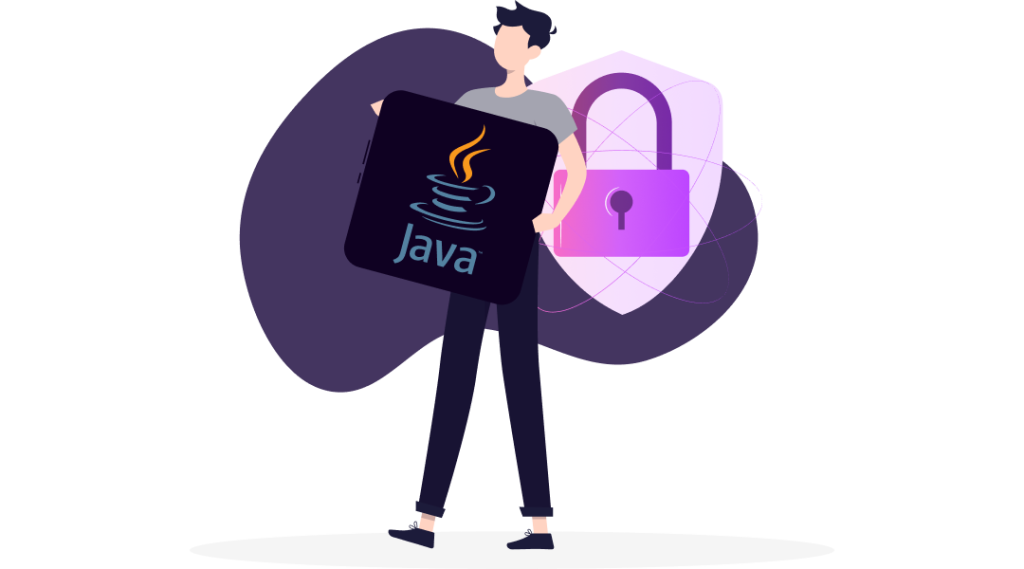
Detecting Licenses and Ensuring Compliance
Java-based projects can pull in dependencies from various open-source repositories. Understanding and adhering to license obligations is crucial to avoid legal and operational risks.
- License Detection
- Leverage tools like LicenseFinder, or License Maven Plugin to scan for license types across dependencies.
- Pay attention to Java-specific licensing, such as Oracle JDK vs. OpenJDK usage and distribution terms.
- Compliance Measures
- Maintain a license compatibility matrix to ensure that combining certain libraries doesn’t violate your organization’s policies.
- Implement automated license scanning in your CI/CD pipeline to prevent merging code that introduces incompatible licenses.
- Flag Critical Licenses
- Permissive Licenses (e.g., Apache 2.0, MIT): Offer fewer restrictions, generally safer for commercial use.
- Restrictive Licenses (e.g., GPL, AGPL): May require open-sourcing your project if combined incorrectly. Understand these obligations thoroughly before use.
Code Ownership and Governance
Proper governance ensures your Java codebase remains maintainable, resilient to turnover, and aligned with best practices over time. Establishing clear code ownership and robust governance structures enables teams to enforce coding standards, streamline decision-making, and promote accountability.
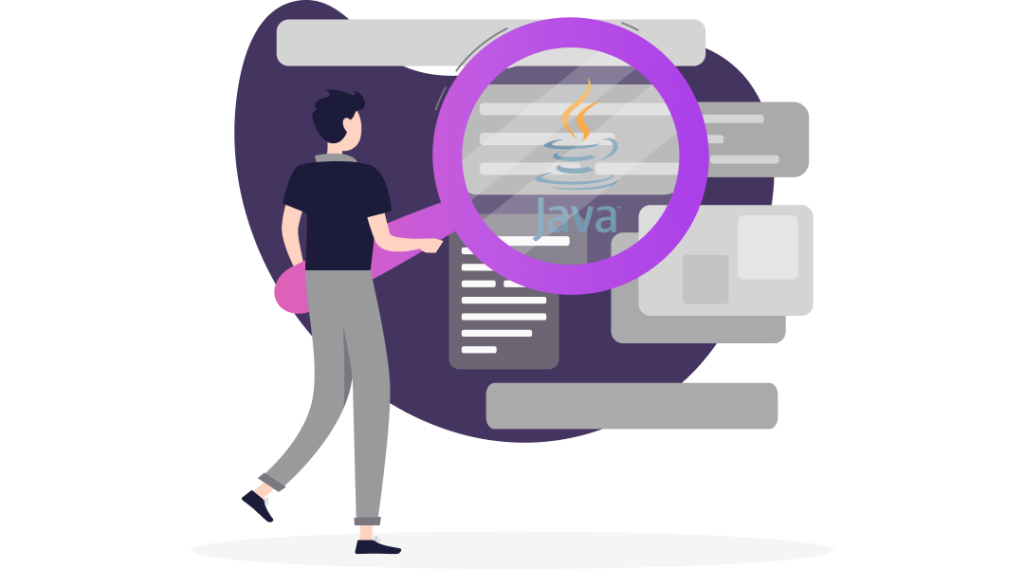
1. Detecting Bad Practices in Code Ownership
Effective code ownership and governance practices keep a project maintainable and resilient to turnover.
1.1 Indicators of Poor Code Ownership
- Ex-Developer Concentration: A large percentage of commits come from inactive contributors, leaving current teams ill-equipped to handle issues.
- Sparse Documentation: Lack of Javadoc, design documentation, or architecture decision records (ADRs).
- Low Codebase Distribution: A small subset of developers are responsible for the majority of the code, creating a “bus factor” risk.
1.2 Code Quality Metrics
- Regularly monitor code coverage (using JaCoCo), complexity (using JavaNCSS), and coding standard compliance (using Checkstyle).
- Analyze commit patterns with git blame or git log to identify potential areas of concern.
2. Tools for Assessment
- Version Control Analysis: Tools like SonarQube can integrate commit data and code quality metrics for deeper insights.
- Code Review Policies: Enforce mandatory peer reviews and track participation to ensure broader knowledge sharing.
3. Mitigation Strategies
3.1 Knowledge Transfer
- Regularly schedule pair programming or mob programming sessions.
- Maintain comprehensive Javadoc and design documentation.
3.2 Code Rotation
- Distribute ownership by rotating module responsibilities.
- Encourage cross-training so multiple developers understand each critical component.
3.3 Monitor Turnover Risks
- Identify “key-person” dependencies and ensure critical areas have more than one qualified maintainer.
Conclusion
Performing a due diligence assessment for Java-based projects involves more than just checking for bugs—it requires a holistic view encompassing security, license compliance, and code governance:
- Security: From input validation and avoiding deserialization attacks to configuring Spring or Hibernate securely and managing dependency risks, staying proactive is paramount.
- License Compliance: Detect and document all licenses to ensure you meet distribution requirements and avoid legal pitfalls.
- Code Ownership & Governance: Encourage balanced contributions, maintain robust documentation, and follow formal code review processes to safeguard knowledge transfer and project continuity.
By integrating these recommendations into regular assessments, you can mitigate risks early, reduce technical debt, and maintain a competitive edge. A well-governed, secure, and legally compliant Java environment forms the backbone of sustainable software development.